Bustabit Script – Manual Enter V2
The Manual Enter Script allows you to easily bet the odds and amount you want without complicated algorithms.
By writing manual bets of different amounts and rates, it will allow you to easily play strategies that normally require very complex coding. You can write your bets and payouts in a list using the “/” sign as a separator. You can check that bets and payouts match correctly by running it in checklist mode before you start. checklist mode is checked by default when the code is first loaded. The first to bet starts with the begin bet and the begin payout. Afterwards, it continues from the list, depending on whether the game is lost or won. When you start the winning series after the lost game, you can play with bet and pay by following the winning list you set. You can run this win list for a certain number of times. “Win game list repetition amount” in the field, enter the number of games you want to play from the list. It is possible to bet by following the same order while switching the win and lose list. By checking the “STARTING POINT SETTING” field, you can continue to bet on the 4th game of your losing list when you lose the 3rd game on the win list. By selecting the “END OF LIST SETTINGS” field, you can repeat the latest bet and payout rates at the end of the list or return to the top of the list.
To help with the continuity of our site and better features, you can donate. Thank you for your support.
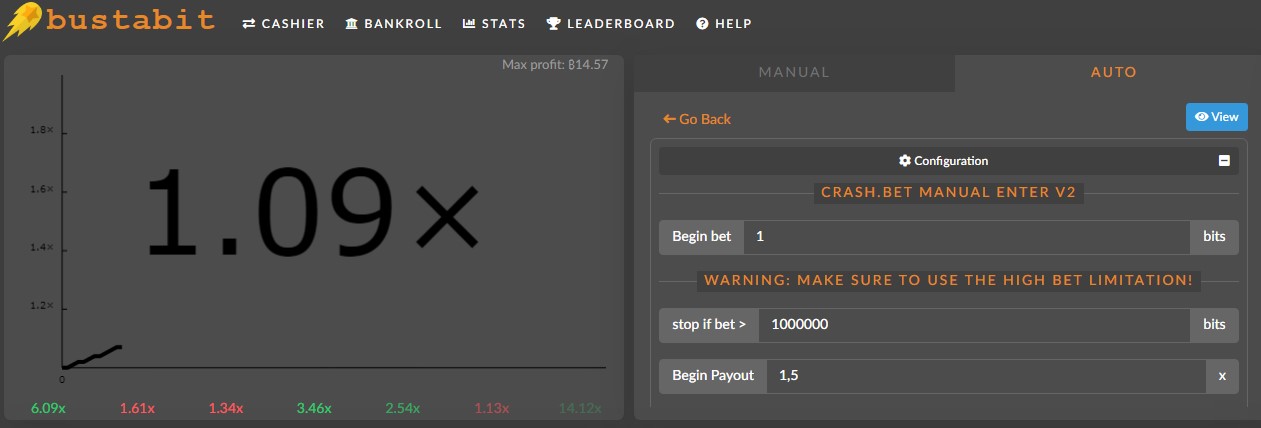
By writing manual bets of different amounts and rates, it will allow you to easily play strategies that normally require very complex coding. You can write your bets and payouts in a list using the “/” sign as a separator. You can check that bets and payouts match correctly by running it in checklist mode before you start. checklist mode is checked by default when the code is first loaded. The first to bet starts with the begin bet and the begin payout. Afterwards, it continues from the list, depending on whether the game is lost or won. When you start the winning series after the lost game, you can play with bet and pay by following the winning list you set. You can run this win list for a certain number of times. “Win game list repetition amount” in the field, enter the number of games you want to play from the list. It is possible to bet by following the same order while switching the win and lose list. By checking the “STARTING POINT SETTING” field, you can continue to bet on the 4th game of your losing list when you lose the 3rd game on the win list. By selecting the “END OF LIST SETTINGS” field, you can repeat the latest bet and payout rates at the end of the list or return to the top of the list.
Watch the case study video.
Change Log
Ver.2.0
- Unlimited manual list entries for bets and payouts.
- List entry for won games.
- Determining the repeat amount for the game won.
- Determining the starting point that follows between won and lost games.
- checklist mode.
How to use script
var config = {
ScriptTitle: {
type: 'noop',
label: ' Crash.Bet Manual Enter v2'
},
baseBet: {
value: 1000,
type: 'balance',
label: 'Begin bet'
},
warning1: {
type: 'noop',
label: 'Warning: Make sure to use the high bet limitation!'
},
stop: {
value: 1e8,
type: 'balance',
label: 'stop if bet >'
},
basePayout: {
value: 2.1,
type: 'multiplier',
label: 'Begin Payout'
},
lossSet: {
type: 'checkbox',
label: 'Lose Game Settings',
value: true
},
lossSetInfo: {
type: 'noop',
label: 'use "/" as separator'
},
lossBets: {
value: '15/20',
type: 'text',
label: 'Lose Bet Series'
},
lossPayouts: {
value: '2.00/1.90',
type: 'text',
label: 'Lose Payout Series'
},
winSet: {
type: 'checkbox',
label: 'Win Game Settings',
value: false
},
winSetInfo: {
type: 'noop',
label: 'use "/" as separator'
},
winBets: {
value: '15/20',
type: 'text',
label: 'Win Bet Series'
},
winPayouts: {
value: '2.00/1.90',
type: 'text',
label: 'Win Payout Series'
},
winBeginSettings: {
value: 'onlyLost',
type: 'radio',
label: 'Won game list setting',
options: {
onlyLost: {
type: "noop",
label: "Play if there is a lost game before"
},
everytime: {
type: 'noop',
label: 'Everytime play the list'
}
}
},
winRepeat: {
value: 0,
type: 'multiplier',
label: 'Win game list repetition amount'
},
startPointTitle: {
type: 'noop',
label: 'Starting point setting'
},
startPoint: {
type: 'checkbox',
label: 'Continue in the same order of the list',
value: false
},
endSettings: {
value: 'turnBack',
type: 'radio',
label: 'End of list settings',
options: {
turnBack: {
type: "noop",
label: "Back to top repeat"
},
contLast: {
type: 'noop',
label: 'Continue from last value'
}
}
},
warning2: {
type: 'noop',
label: 'Check the list before starting the game!'
},
checkList: {
type: 'checkbox',
label: 'Check List Mode (Print values for check)',
value: true
}
};
log('Script is running..');
var currentBet = config.baseBet.value;
var currentPayout = config.basePayout.value
var lossBetSeriesRaW = config.lossBets.value
var lossBetSeries = lossBetSeriesRaW.split("/")
var lossPayoutSeriesRaw = config.lossPayouts.value
var lossPayoutSeries = lossPayoutSeriesRaw.split("/")
var winBetSeriesRaw = config.winBets.value
var winBetSeries = winBetSeriesRaw.split("/")
var winPayoutSeriesRaw = config.winPayouts.value
var winPayoutSeries = winPayoutSeriesRaw.split("/")
var gameCount = 0
var lossGameCount = 0
var winGameCount = 0
var shiftGameCount = 0
var repValidCount = 0
var lossStatus = false
if (config.checkList.value) {
checkList()
} else {
if (config.lossSet.value) {
if (lossBetSeries.length != lossPayoutSeries.length) {
stop('Bets and payouts do not matched, bets and payouts must be the same amount.')
}
}
if (config.winSet.value) {
if (winBetSeries.length != winPayoutSeries.length) {
stop('Bets and payouts do not matched, bets and payouts must be the same amount.')
}
}
engine.bet(roundBit(currentBet), currentPayout)
log('Begining game Bet:', currentBet / 100, 'bits, Payout', currentPayout)
engine.on('GAME_STARTING', onGameStarted)
engine.on('GAME_ENDED', onGameEnded)
}
function onGameStarted() {
engine.bet(roundBit(currentBet), roundPo(currentPayout));
log('Bet was made, Bet: ', roundBit(currentBet) / 100, 'bits, Payout: ', roundPo(currentPayout))
}
function onGameEnded() {
var lastGame = engine.history.first()
if (!lastGame.wager) {
return;
}
if (lastGame.cashedAt) {
if (config.startPoint.value) {
gameCount = shiftGameCount
} else {
gameCount = winGameCount
}
if (!config.winSet.value) {
currentBet = config.baseBet.value;
currentPayout = config.basePayout.value
} else {
if (config.winBeginSettings.value === 'onlyLost' && lossStatus) {
if (gameCount >= winBetSeries.length) {
if (config.endSettings.value === 'contLast') {
currentBet = parseFloat(winBetSeries[winBetSeries.length - 1]) * 100
currentPayout = parseFloat(winPayoutSeries[winBetSeries.length - 1])
} else {
winGameCount = 0
shiftGameCount = 0
if (config.startPoint.value) {
gameCount = shiftGameCount
} else {
gameCount = winGameCount
}
currentBet = parseFloat(winBetSeries[gameCount]) * 100
currentPayout = parseFloat(winPayoutSeries[gameCount])
}
} else {
currentBet = parseFloat(winBetSeries[gameCount]) * 100
currentPayout = parseFloat(winPayoutSeries[gameCount])
}
} else {
if (config.winBeginSettings.value === 'everytime') {
if (gameCount >= winBetSeries.length) {
if (config.endSettings.value === 'contLast') {
currentBet = parseFloat(winBetSeries[winBetSeries.length - 1]) * 100
currentPayout = parseFloat(winPayoutSeries[winBetSeries.length - 1])
} else {
winGameCount = 0
shiftGameCount = 0
if (config.startPoint.value) {
gameCount = shiftGameCount
} else {
gameCount = winGameCount
}
currentBet = parseFloat(winBetSeries[gameCount]) * 100
currentPayout = parseFloat(winPayoutSeries[gameCount])
}
} else {
currentBet = parseFloat(winBetSeries[gameCount]) * 100
currentPayout = parseFloat(winPayoutSeries[gameCount])
}
} else {
currentBet = config.baseBet.value
currentPayout = config.basePayout.value
}
}
}
log('You Win, Next game Bet: ', roundBit(currentBet) / 100, 'bits, Payout: ', roundPo(currentPayout))
shiftGameCount++
winGameCount++
repValidCount++
lossGameCount = 0
if (repValidCount >= config.winRepeat.value) {
lossStatus = false
}
} else {
if (config.startPoint.value) {
gameCount = shiftGameCount
} else {
gameCount = lossGameCount
}
if (!config.lossSet.value) {
currentBet = config.baseBet.value;
currentPayout = config.basePayout.value
} else {
if (gameCount >= lossBetSeries.length) {
if (config.endSettings.value === 'contLast') {
currentBet = parseFloat(lossBetSeries[lossBetSeries.length - 1]) * 100
currentPayout = parseFloat(lossPayoutSeries[lossBetSeries.length - 1])
} else {
lossGameCount = 0
shiftGameCount = 0
if (config.startPoint.value) {
gameCount = shiftGameCount
} else {
gameCount = lossGameCount
}
currentBet = parseFloat(lossBetSeries[gameCount]) * 100
currentPayout = parseFloat(lossPayoutSeries[gameCount])
}
} else {
currentBet = parseFloat(lossBetSeries[gameCount]) * 100
currentPayout = parseFloat(lossPayoutSeries[gameCount])
}
}
log('You Loss, Next game Bet: ', roundBit(currentBet) / 100, 'bits, Payout: ', roundPo(currentPayout))
shiftGameCount++
lossGameCount++
winGameCount = 0
repValidCount = 0
lossStatus = true
}
if (currentBet > config.stop.value) {
engine.removeListener('GAME_STARTING', onGameStarted)
engine.removeListener('GAME_ENDED', onGameEnded)
stop(currentBet, 'Maximum amount reached, Script stopped.')
}
}
function roundBit(bet) {
return Math.round(bet / 100) * 100;
}
function roundPo(payout) {
return Math.round(payout * 100) / 100;
}
function checkList() {
var i
log('----------CHECK LIST----------')
log('First Game, Base Bet: ', roundBit(currentBet) / 100, ' Base Payout: ', currentPayout)
if (config.lossSet.value) {
log('Loss game list mode on')
if (lossBetSeries.length === lossPayoutSeries.length) {
log('Bets and payouts matched')
for (i = 0; i < lossBetSeries.length; i++) {
log('Game', i + 1, ' Bet: ', lossBetSeries[i], ' Payout: ', lossPayoutSeries[i])
}
} else {
log('Bets and payouts do not matched, bets and payouts must be the same amount.')
}
} else {
log('Loss game list mode off')
}
if (config.winSet.value) {
log('Win game list mode on')
if (winBetSeries.length === winPayoutSeries.length) {
log('Bets and payouts matched')
for (i = 0; i < winBetSeries.length; i++) {
log('Game', i + 1, ' Bet: ', winBetSeries[i], ' Payout: ', winPayoutSeries[i])
}
} else {
log('Bets and payouts do not matched other, bets and payouts must be the same amount.')
}
} else {
log('Win game list mode off')
}
log('Begin of list mode: ', config.winBeginSettings.value === 'onlyLost' ? 'Play if there is a lost game before' : 'Everytime play the list')
log('End of list mode: ', config.endSettings.value === 'turnBack' ? 'Back to top repeat' : 'Continue from last value')
log('Continue of list mode: ', config.startPoint.value ? 'Continue from the game in the same order of the list' : 'Play from the top of the list')
log('If there is a lost game in the game before the winning match, plays ', config.winRepeat.value, ' games from the win game list.')
log('(Available only when "Play if game lost before starting mode is set" option is selected.)')
log(' ')
stop('End of check list')
}
To help with the continuity of our site and better features, you can donate. Thank you for your support.
| #bustabit autobet | #bustabit bot | #bustabit cheat | #bustabit crash predictor | #bustabit crash script | #bustabit crash strategy | #bustabit hack script | #bustabit script | #bustabit strategy | #wining script