BC.Game Script - Manual Enter V3
The Manual Enter Script allows you to easily bet the odds and amount you want without complicated algorithms.
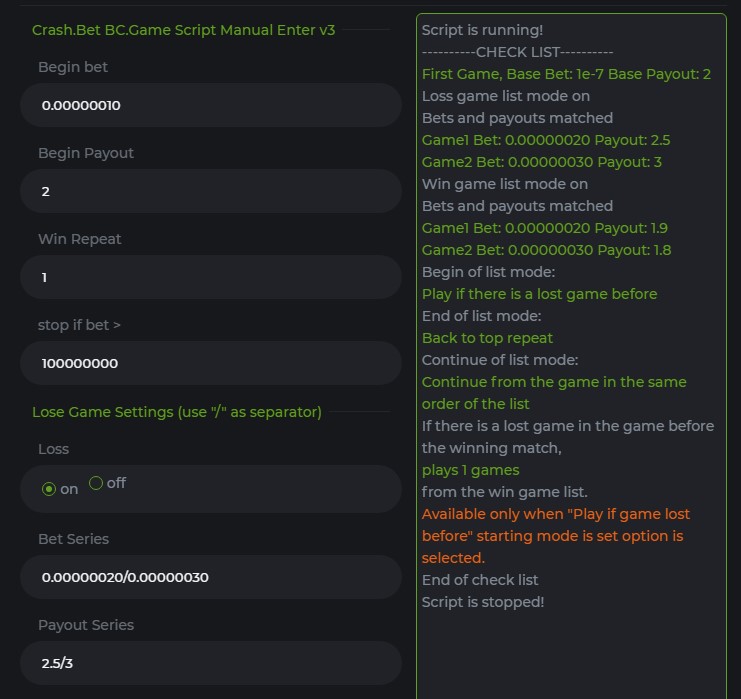
By writing manual bets of different amounts and rates, it will allow you to easily play strategies that normally require very complex coding. You can write your bets and payouts in a list using the "/" sign as a separator. You can check that bets and payouts match correctly by running it in checklist mode before you start. checklist mode is checked by default when the code is first loaded. The first to bet starts with the begin bet and the begin payout. Afterwards, it continues from the list, depending on whether the game is lost or won. When you start the winning series after the lost game, you can play with bet and pay by following the winning list you set. You can run this win list for a certain number of times. "Win game list repetition amount" in the field, enter the number of games you want to play from the list. It is possible to bet by following the same order while switching the win and lose list. By checking the "STARTING POINT SETTING" field, you can continue to bet on the 4th game of your losing list when you lose the 3rd game on the win list. By selecting the "END OF LIST SETTINGS" field, you can repeat the latest bet and payout rates at the end of the list or return to the top of the list.
Watch the case study video.
Change Log
Ver.3.0
- Code update for BC.Game V3.
- Correction of typos in the interface and console notifications.
Ver.2.0
- Unlimited manual list entries for bets and payouts.
- List entry for won games.
- Determining the repeat amount for the game won.
- Determining the starting point that follows between won and lost games.
- checklist mode.
How to use script
var config = {
ScriptTitle: {
label: 'Crash.Bet BC.Game Script Manual Enter v3',
type: 'title'
},
baseBet: {
value: currency.minAmount,
type: 'number',
label: 'Begin bet'
},
basePayout: {
value: 2,
type: 'number',
label: 'Begin Payout'
},
winRepeat: {
value: 1,
type: 'number',
label: 'Win Repeat'
},
stop: {
value: 1e8,
type: 'number',
label: 'stop if bet >'
},
loseSetTitle: {
label: 'Lose Game Settings (use "/" as separator)',
type: 'title'
},
lossSeriesSet: {
value: 0,
type: 'radio',
label: 'Loss',
options: [{
value: 1,
label: 'on'
},
{
value: 0,
label: 'off'
}
]
},
lossBetSeries: {
value: '2/3',
type: 'text',
label: 'Bet Series'
},
lossPoSeries: {
value: '2.5/3',
type: 'text',
label: 'Payout Series'
},
winSetTitle: {
label: 'Win Game Settings (use "/" as separator)',
},
winSeriesSet: {
value: 0,
type: 'radio',
label: 'Win',
options: [{
value: 1,
label: 'on'
},
{
value: 0,
label: 'off'
}
]
},
winBetSeries: {
value: '2/3',
type: 'text',
label: 'Bet Series'
},
winPoSeries: {
value: '1.9/1.8',
type: 'text',
label: 'Payout Series'
},
beginSetTitle: {
label: 'Begin Series Settings',
type: 'title'
},
beginSeriesSet: {
value: 1,
type: 'radio',
label: 'Begin Series Setting',
options: [{
value: 1,
label: "Play if there is a lost game before"
},
{
value: 0,
label: 'Everytime play the list'
}
]
},
continueSetTitle: {
label: 'Continue Series Settings',
type: 'title'
},
continueSeriesSet: {
value: 1,
type: 'radio',
label: 'Continue Series Setting',
options: [{
value: 1,
label: 'Continue in the same order of the list',
},
{
value: 0,
label: 'Continue in the top of the list',
}
]
},
endSetTitle: {
label: 'End of Series Setting',
type: 'title'
},
endSeriesSet: {
value: 1,
type: 'radio',
label: 'End of Series Setting',
options: [{
value: 1,
label: "Back to top repeat"
},
{
value: 0,
label: 'Continue from last value'
}
]
},
checkListTitle: {
label: 'Check List Mode',
type: 'title'
},
checkListMode: {
value: 1,
type: 'radio',
label: 'Check List Mode',
options: [{
value: 1,
label: 'on'
},
{
value: 0,
label: 'off'
}
]
}
};
function main() {
var beginBet = config.baseBet.value
var beginPayout = config.basePayout.value
var currentBet = beginBet
var currentPayout = beginPayout
var lossSeriesSet = config.lossSeriesSet.value
var lossBetSeries = config.lossBetSeries.value.split("/")
var lossPoSeries = config.lossPoSeries.value.split("/")
var winSeriesSet = config.winSeriesSet.value
var winBetSeries = config.winBetSeries.value.split("/")
var winPoSeries = config.winPoSeries.value.split("/")
var winRepeat = config.winRepeat.value
var beginStatus = config.beginSeriesSet.value
var contStatus = config.continueSeriesSet.value
var endStatus = config.endSeriesSet.value
var checkModeStatus = config.checkListMode.value
var lossStatus = 0
var winStatus = 0
var baseStatus = 1
var gameCount = 0
var repProvision = 0
var repValidCount = 0
var matchValidEquals = 0
var matchValidGreater = 0
var matchValidLess = 0
if (checkModeStatus) {
checkList()
game.stop();
return
}
matchCheckGreaterEqualsLess(lossBetSeries.length, lossPoSeries.length)
if (matchValidEquals) {
matchValidRestore()
} else {
matchValidRestore()
log.error('Bets and payouts do not matched, bets and payouts must be the same amount.')
game.stop();
return
}
game.onBet = function () {
log.success('Game Starting, Bet: ' + currentBet + 'Payout: '+ currentPayout)
game.bet(currentBet, currentPayout).then(function (payout) {
// Win
if (payout > 1) {
if (!contStatus) {
if (!winStatus) {
counterReset()
}
} else {
if (!beginStatus) {
if (lossStatus) {
lossStatus--
}
}
}
if (!winStatus) {
winStatus++
}
if (baseStatus) {
baseStatus--
counterReset()
}
if (!winSeriesSet) {
gameStarter(beginBet, beginPayout)
if (!baseStatus) {
baseStatus++
}
} else {
if (beginStatus && lossStatus) {
matchCheckGreaterEqualsLess(gameCount, winBetSeries.length)
if (matchValidEquals || matchValidGreater) {
matchValidRestore()
if (!endStatus) {
gameStarter(parseFloat(winBetSeries[winBetSeries.length - 1]), parseFloat(winPoSeries[winBetSeries.length - 1]))
} else {
counterReset()
gameStarter(parseFloat(winBetSeries[gameCount]), parseFloat(winPoSeries[gameCount]))
}
} else {
matchValidRestore()
gameStarter(parseFloat(winBetSeries[gameCount]), parseFloat(winPoSeries[gameCount]))
}
} else {
if (!beginStatus) {
matchCheckGreaterEqualsLess(gameCount, winBetSeries.length)
if (matchValidEquals || matchValidGreater) {
matchValidRestore()
if (!endStatus) {
gameStarter(parseFloat(winBetSeries[winBetSeries.length - 1]), parseFloat(winPoSeries[winBetSeries.length - 1]))
} else {
counterReset()
gameStarter(parseFloat(winBetSeries[gameCount]), parseFloat(winPoSeries[gameCount]))
}
} else {
matchValidRestore()
gameStarter(parseFloat(winBetSeries[gameCount]), parseFloat(winPoSeries[gameCount]))
}
} else {
gameStarter(beginBet, beginPayout)
if (!baseStatus) {
baseStatus++
}
}
}
}
log.success('You Win!!')
gameCount++
repValidCount++
repProvision--
matchCheckGreaterEqualsLess(repValidCount, winRepeat)
if (matchValidEquals || matchValidGreater) {
matchValidRestore()
if (lossStatus) {
lossStatus--
}
}
} else {
// Loss
if (!contStatus) {
if (winStatus) {
counterReset()
}
}
if (!lossStatus) {
lossStatus++
}
if (winStatus) {
winStatus--
}
if (baseStatus) {
baseStatus--
counterReset()
}
if (!lossSeriesSet) {
gameStarter(beginBet, beginPayout)
if (!baseStatus) {
baseStatus++
}
} else {
matchCheckGreaterEqualsLess(gameCount, lossBetSeries.length)
if (matchValidEquals || matchValidGreater) {
matchValidRestore()
if (!endStatus) {
gameStarter(parseFloat(lossBetSeries[lossBetSeries.length - 1]), parseFloat(lossPoSeries[lossBetSeries.length - 1]))
} else {
counterReset()
gameStarter(parseFloat(lossBetSeries[gameCount]), parseFloat(lossPoSeries[gameCount]))
}
} else {
matchValidRestore()
gameStarter(parseFloat(lossBetSeries[gameCount]), parseFloat(lossPoSeries[gameCount]))
}
log.error('You Loss')
gameCount++
winRepCountReset()
}
}
})
}
function gameStarter(calculatedBet, calculatedPayout) {
if (currentBet > config.stop.value) {
log.error('Maximum amount reached, Script stopped.');
game.stop();
}
log.info('betting')
currentBet = calculatedBet
currentPayout = calculatedPayout.toFixed(2)
}
function checkList() {
var i
log.info('----------CHECK LIST----------')
log.success('First Game, Base Bet: ' + beginBet + ' Base Payout: ' + beginPayout)
if (lossSeriesSet) {
log.info('Loss game list mode on')
matchCheckGreaterEqualsLess(lossBetSeries.length, lossPoSeries.length)
if (matchValidEquals) {
matchValidRestore()
log.info('Bets and payouts matched')
seriesList(lossBetSeries, lossPoSeries)
} else {
matchValidRestore()
log.error('Bets and payouts do not matched, bets and payouts must be the same amount.')
}
} else {
log.error('Loss game list mode off')
}
if (winSeriesSet) {
log.info('Win game list mode on')
matchCheckGreaterEqualsLess(winBetSeries.length, winPoSeries.length)
if (matchValidEquals) {
matchValidRestore()
log.info('Bets and payouts matched')
seriesList(winBetSeries, winPoSeries)
} else {
matchValidRestore()
log.error('Bets and payouts do not matched other, bets and payouts must be the same amount.')
}
} else {
log.error('Win game list mode off')
}
log.info('Begin of list mode:')
log.success(beginStatus ? 'Play if there is a lost game before' : 'Everytime play the list')
log.info('End of list mode:')
log.success(endStatus ? 'Back to top repeat' : 'Continue from last value')
log.info('Continue of list mode:')
log.success(contStatus ? 'Continue from the game in the same order of the list' : 'Play from the top of the list')
log.info('If there is a lost game in the game before the winning match,')
log.success('plays ' + winRepeat + ' games ')
log.info('from the win game list.')
log.error('Available only when "Play if game lost before" starting mode is set option is selected.')
log.info('End of check list')
}
function counterReset() {
if (!gameCount) {
return
}
gameCount--
counterReset()
}
function winRepCountReset() {
if (!repProvision) {
return
}
repValidCount--
repProvision++
if (!repProvision) {
return
}
winRepCountReset()
}
function matchCheckGreaterEqualsLess(listOne, listTwo) {
if (!listOne || !listTwo) {
if (!listTwo && !listOne) {
matchValidEquals++
return
} else {
if (!listOne) {
matchValidLess++
return
} else {
matchValidGreater++
return
}
}
}
listOne--
listTwo--
matchCheckGreaterEqualsLess(listOne, listTwo)
}
function matchValidRestore() {
if (matchValidEquals) {
matchValidEquals--
}
if (matchValidGreater) {
matchValidGreater--
}
if (matchValidLess) {
matchValidLess--
}
}
}
function seriesList(listOne, listTwo) {
listLenghtIdentfy(listOne.length, listTwo.length, 1)
function listLenghtIdentfy(listOneLength, listTwoLenght, listCount) {
log.success('Game' + listCount + ' Bet: ' + listOne[listCount - 1] + ' Payout: ' + listTwo[listCount - 1])
listOneLength--
listTwoLenght--
listCount++
if (!listOneLength) {
return
}
listLenghtIdentfy(listOneLength, listTwoLenght, listCount)
}}
To help with the continuity of our site and better features, you can donate. Thank you for your support.
| #autobet | #bc game bot | #bc game cheat | #bc game crash predictor | #bc game crash script | #bc game crash strategy | #bc game hack script | #bc game script | #bc.game | #bc.game script